we call parent class as ‘super class’ , ‘base class’
and child class as ‘subclass’ , ‘derived class'
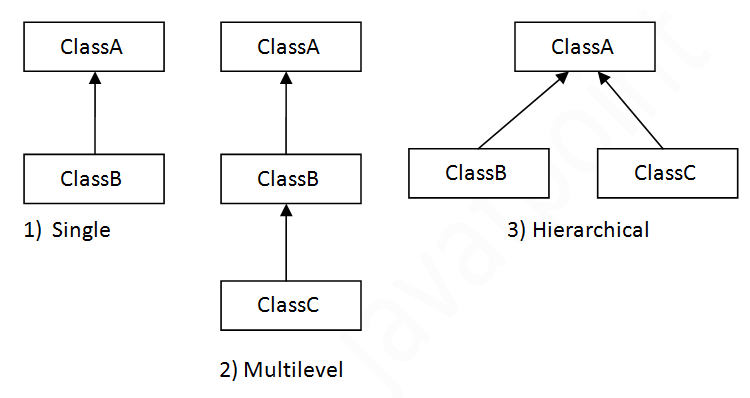
express as above picture (ClassA is super class)
Java ingeritance
inheritance by using extend keyword
exampe)Python inheritance
Customer.java
package inheritance;
public class Customer{
protected int customerID;
protected String customerName;
protected String customerGrade;
int bonusPoint;
double bonusRatio;
public Customer(){
customerGrade="SILVER";
bonusRatio=0.01;
}
public int calcPrice(int price){
bonusPoint+=price*bonusRatio;
return price;
}
public String showCustomerInfo(){
return customerName+"님의 등급은" +customerGrade+"이며, 보너스 포인트는"+bonusPoint+"입니다.";
}
public int getCustomerID(){
return customerID;
}
public void setCustomerID(int customerID){
this.customerID=customerID;
}
public String getCustomerName(){
return customerName;
}
public void setCustomerName(String customerName){
this.customerName=customerName;
}
public String getCustomerGrade(){
return customerGrade;
}
public void setCustomerGrade(String customerGrade){
this.custoemrGrade=customerGrade;
}
}
VIPCustomer.java
package ingeritacne;
public class VIPCustomer extends Customer{
private int agentID;
double saleRatio;
public VIPCustomer(){
customerGrade="VIP";
bonusRatio=0.05;
saleRatio=0.1;
}
public int getAgentID(){
return agentID;
}
@Override
public int calcPrice(int price){
bonus+=price*bonusRatio;
return price-(int)(price*saleRatio);
}
}
CustomerTest1.java
package inheritance;
public class CustomerTest1{
public static void main(String[] args){
Customer customerLee=new Customer();
customerLee.setCustomerID(10010);
customerLee.setCustomerName("이순신");
customerLee.bonusPoint=1000;
System.out.println(customerLee.showCustomerInfo());
VIPCustomer customerKim=new VIPCustomer();
customerKim.setCustomerID(10020);
customerKim.setCustomerName("김유신");
customerKim.bonusPoint=10000;
System.out.println(customerKim.showCustomerInfo());
}
}
Super
super is calling parent class keywords
before using child class must call parent class first.
if parent class do not have default constructor(생성자) at child class must call parent class constructor.
public VIPCUstomer(int customerID,String customerName,int agentID){
super(customerID,customerName);
customerGrade="VIP";
bonusRatio=0.05;
saleRatio=0.1;
this.agentID=agentID;
}
Annotation(주석)
'@AnnotationName’
Annotation |
explain |
@Override |
재정의된 메서드라는 정보 제공 |
@FuctionalInterface |
함수형 인터페이스라는 정보 제공 |
@Deprecated |
이후 버전에서 사용되지 않을 수 있는 변수, 메서드에 사용됨 |
@SuppressWarnings |
특정 경고가 나타나지 않도록 함 |
ect...
Virtual method
package inheritance;
public class OverridingTest3{
public static void main(String[] args){
int price = 10000;
Customer customerLee=new VIPCustomer(10010,"이순신");
System.out.println(customerLee.calcPrice(price));
}
}
because of virtual method
customerLee의 자료형은 Customer 이지만 calcPrice()가 호출되면,
Customer의 메서드가 호출되는 것이 아닌 인스턴스(VIPCustomer)의 메서드가 호출됨.